How do I count the lines of a text object? I want to change the font size if a text object is too many lines…
I’m assuming you were asking for a basic feature that would do this, but unfortunately there isn’t really anything like that. On the other hand I did create an algorithm to do this for you, be warned it is a little complex.
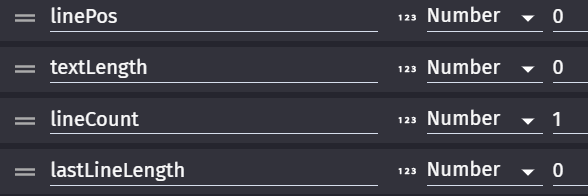
You can change the 4 to however many lines you want to look for before the font changes. lineCount starts at one because we assume there will always be at least one line.
The way it works is that it searches for newLine() characters and increments the lineCount number until the second to last line is reached (there is no newLine() at the very end of a String), hence why lineCount starts at one. That’s also why the condition is linePos - lastLineLength.
I’m making a clicker game, and I want to make big numbers smaller, so when the text is multiple lines, it decreases. I tried to implement your code, but it only works when I do f(x)Variable(linecount)=1, not when it is a higher value. “Flags” is the text object.
This is my code:
The code that I’m using to make the text multiple lines is: when space bar is released, change text of flags set to 100000000.
Thanks so much!
Can you post a screenshot of what the text object looks like with the text in it and another of what it looks like after it is changed? This is what I was using as a test
If the text is changing at runtime then it would probably be best to instead of having “at the beginning of the scene” to change the text length and last line length, instead just make it have no condition (this is just for testing).
Ideally, it might be best to run this algorithm after the space bar has been pressed, as like a sub event.
I kept trying to fix it but it didn’t work, so I just came up with another option: I check if a certain digit (0-9) is in the number of “cookies,” and then add a certain percentage (font size divided by the smallest possible width of the text object). Then, if the percentage exceeded a certain amount (the sum of the widest digits without making two lines), it would reduce the font size by 90. It’s like checking how many digits are in a line, but a lot easier. Thanks for the help anyway!
Great solution, I wish I would have known you were using numbers I would have suggested something just like that
Thanks! I’ll keep that in mind and add more details next time I ask a question.
I am having one more issue - I can determine if a number exists in a string–for example, “1” is in the number “1,000.” However, I can’t count all of the instances. For example, how many 0s are in “1,000.”
Thanks in advance!
Here you go, this algorithm is quite simple. It checks each character of a string, if the character is 0 a variable zeroCounter is incremented by one and the index is incremented by one to the next character. If there is no 0 then the index is incremented again by one but zeroCounter is not incremented. By the time the index reaches the text’s length, the zeroCounter will have the exact number of zeros.